|
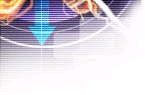 |
PHP...
a powerful, flexible, fully supported, battle tested server side language .. |
|
|
|
 | PHP > What are Functions in PHP? |  |
Functions are essential building blocks in PHP programming, allowing you to encapsulate logic, promote code reuse, and improve code organization. By understanding how to define, call, and work with functions, you'll be able to write more modular and maintainable PHP code.
Functions in PHP are blocks of reusable code that perform a specific task. They allow you to break down your code into smaller, manageable parts, making it easier to read, understand, and maintain.
 | Creating a Function |  |
To create a function in PHP, you use the `function` keyword followed by the function name and parentheses containing any parameters the function accepts. Here's a basic example:
|
|