|
|
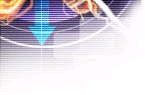 |
PHP...
a powerful, flexible, fully supported, battle tested server side language .. |
|
|
|
 | Secure Login (PHP) |  |
When developing a robut and secure login - you need to think outside the box - you also need to think of 'human' error - using redundant checks (layers of checks).
For example:
• No Single Point of Failure: Compromising one layer (e.g., IP spoofing) doesn't bypass others (device fingerprinting, behavioral checks).
• Adaptive Security: Legitimate user factors; attackers hit escalating barriers.
• Future-Proofing: Combats AI-driven attacks (e.g., bots and AI systems monitoring data or individuals to try and bypass any security).
 | Basic Security Components |  |
Authentication • Force https
• Username & Password
• All inputs are cleaned and checked - regular expressions, maximum length
• Dynamic Digit Challenge - Randomly asks for different digits each login.
• Zero-Knowledge Proofs (ZKPs) - Similar to dynamic digit but allows users to prove they know their security number without revealing the digits - e.g., 2nd digit times the 3rd digit + 3 equals? - only give single number reply
• Captcha Number - generate image of a number (or colors or shapes)
• IP history - email OTP - 'unknown' IP require multifactor authentication (confirmation from number sent to email) - time limited (30 minutes)
• If IP isn't used in over 20 days - it's automatically deleted (need to confirm identity if logged in from this IP after 20 days)
• SQLITE (track login activity/history/data and information)
- database should block/prevent any database attacks/patterns
• Log - each user can see their past login history (e.g., valid logins, any failed login attempts on their account) - history of access to their account - see if anyone has been trying to get access.
• Login Page Tokenization - Generate one-time tokens for login forms (Prevents CSRF)
• Rate Limiting with Jails: Lock account after 5 digit/OTP failures (either 'ban' or block for x hours)
• Honeypot: Fake form fields to trap bots.
• Decoy Accounts & Fake Login Paths - hidden link on login page - if link is accessed block IP (link cannot be seen - only be accessed by looking at the html - -trap)
- try logging in using known hacks - like 'user' 'pass' it identifies as hack/attack - blocks IP
- url traps
|
|
|
|
|
|