|
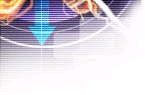 |
PHP...
a powerful, flexible, fully supported, battle tested server side language .. |
|
|
|
 | PHP > PHP Strings and Syntax Basics |  |
Strings are very very very very very important for the web - everything from generating web-page content to parsing input from users. But not to worry, PHP provides a rich set of functions for working with strings, from basic operations like concatenation to more advanced tasks like pattern matching with regular expressions. Understanding these functions and their usage will empower you to manipulate strings effectively in your PHP projects. Remember to always validate user input and handle edge cases appropriately for robust and secure code.
In PHP, a string is a sequence of characters, like "Hello, World!". You can use single quotes ('') or double quotes ("") to define strings.
|
|