|
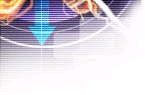 |
Fractals
Natures pattern... |
|
|
|
Fractal patterns, including XOR and plasma, are captivating visual phenomena that emerge from the iterative application of simple mathematical rules. These patterns exhibit self-similarity, meaning that they appear similar at different scales, offering intricate and mesmerizing detail when zoomed in or out. Fractals have found applications in various fields, from art and design to science and engineering, owing to their aesthetic appeal and underlying mathematical elegance.
 | XOR Fractal Pattern |  |
| |